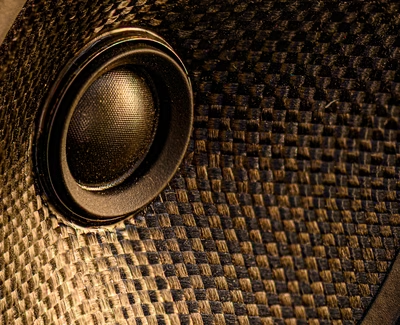
Texto a voz de Google
Uso de Google Text-to-Speech con Python sin guardar en un archivo.
El motor de Google Text-to-Speech sigue siendo, con mucho, la mejor y más natural voz disponible en el mercado. Si bien hay muchos ejemplos de cómo usar la biblioteca TTS con Python, todos asumen que desea guardar el clip de audio resultante en un archivo .mp3 para poder reproducirlo.
Guardar el archivo para reproducir el sonido y luego eliminarlo no parece gran cosa, pero en una Raspberry Pi usando una tarjeta SD con un número limitado de ciclos de lectura y escritura, simplemente no parece una gran idea. Por lo tanto, estaba buscando una solución para reproducir la transmisión de audio, y esta es la forma más confiable que encontré para hacerlo.
Este código supone que ya se ha registrado en el servicio Google Text-to-Speech y que ha obtenido el archivo GOOGLE_APPLICATION_CREDENTIALS .json adecuado. Consulte la documentación de Google sobre cómo hacerlo.
Las bibliotecas de Pygame también se utilizarán para ejecutar este script, consulte también su documentación sobre cómo instalarlo en su sistema.
from google.cloud import texttospeech
import pygame
import io
import os
os.putenv('DISPLAY', ':0.0')
os.environ["SDL_VIDEODRIVER"] = "dummy"
os.environ["GOOGLE_APPLICATION_CREDENTIALS"] = os.path.expanduser("~/<your_credential_file>.json")
# Instantiates a client
client = texttospeech.TextToSpeechClient()
# response = client.list_voices()
# print(response)
# Set the text input to be synthesized
synthesis_input = texttospeech.types.SynthesisInput(text="Knock knock! Why did the duck cross the road?")
# Build the voice request, select the language code ("en-US") and the ssml
voice = texttospeech.types.VoiceSelectionParams(
language_code='en-US',
name='en-US-Wavenet-F',
ssml_gender=texttospeech.enums.SsmlVoiceGender.FEMALE)
# Select the type of audio file you want returned
audio_config = texttospeech.types.AudioConfig(
audio_encoding=texttospeech.enums.AudioEncoding.MP3)
# Perform the text-to-speech request on the text input with the selected
# voice parameters and audio file type
response = client.synthesize_speech(synthesis_input, voice, audio_config)
# # The response's audio_content is binary.
# with open('output.mp3', 'wb') as out:
# # Write the response to the output file.
# out.write(response.audio_content)
# print('Audio content written to file "output.mp3"')
freq = 24000 # audio CD quality
bitsize = -16 # unsigned 16 bit
channels = 2 # 1 is mono, 2 is stereo
buffer = 2048 # number of samples (experiment to get right sound)
pygame.mixer.init(freq, bitsize, channels, buffer)
pygame.mixer.music.set_volume(1.0)
pygame.display.set_mode((1, 1))
pygame.mixer.init()
pygame.init() # this is needed for pygame.event.* and needs to be called after mixer.init() otherwise no sound is played
with io.BytesIO() as f: # use a memory stream
f.write(response.audio_content)
f.seek(0)
pygame.mixer.music.load(f)
pygame.mixer.music.set_endevent(pygame.USEREVENT)
pygame.event.set_allowed(pygame.USEREVENT)
pygame.mixer.music.play()
pygame.event.wait() # play() is asynchronous. This wait forces the speaking to be finished before closing f and returning
while pygame.mixer.music.get_busy():
pygame.time.Clock().tick(10)
pygame.mixer.music.fadeout(1000)
pygame.mixer.music.stop()
Tagged with:
text-to-speech